JAVA
Monday, January 08, 2007
Monday, December 25, 2006
Wednesday, December 20, 2006
Monday, December 18, 2006
Sunday, December 10, 2006
Lab Temperature Project
package abb1;
public class Temperature
{
private double degree;
private char scale;
public Temperature()
{
this.degree=0;
this.scale='c';
}
public Temperature(double degree)
{
this.degree=degree;
this.scale='c';
}
public Temperature(char scale)
{
this.degree=0;
if(scale=='f'||scale=='F')
{
this.scale='F';
}
else if(scale=='c'||scale=='C')
{
this.scale='C';
}
else
{
System.out.println("error scale and please scale set C");
this.scale='C';
}}
public Temperature(double degree,char scale)
{
this.degree=degree;
if(scale=='f'||scale=='F')
{
this.scale='F';
}
else if(scale=='c'||scale=='C')
{
this.scale='C';
}
else
{
System.out.println("error scale and please scale set C");
this.scale='C';
}}
public double get_temperatureC()
{
double degreesC=0;
if(scale=='C')
{
degreesC=degree;
}
else if(scale=='F')
{
degreesC = (degree -32 )*5/9;
}
return degreesC;
}
public double get_temperatureF()
{
double degreesF=0;
if(scale=='F')
{
degreesF=degree;
}
else if(scale=='C')
{
degreesF = (degree *9/5 )+32;
}
return degreesF;
}
public void setdegree(double degree)
{
this.degree=degree;
}
public void setScale(char scale)
{
if(scale=='f'||scale=='f')
{
this.scale='F';
}
else if(scale=='c'||scale=='C')
{
this.scale='C';
}
else
{
System.out.println("Error scale and scale no change");
}}
public void setScale(double degree,char scale)
{
if(scale=='f'||scale=='f')
{
this.scale='F';
}
else if(scale=='c'||scale=='C')
{
this.scale='C';
}
else
{
System.out.println("Error scale and scale no change");
}
this.degree=degree;
}
public boolean isequals(Temperature othertemp)
{
if (this.scale == 'C' && this.degree == othertemp.get_temperatureC())
{
return true;
}
else if (this.scale == 'F' && this.degree == othertemp.get_temperatureF())
{
return true;
}
else
{
return false;
}}
public boolean isgreaterthan(Temperature otherTemp)
{
if (this.scale == 'C' && this.degree >= otherTemp.get_temperatureC())
{
return true;
}
else if (this.scale == 'F' && this.degree >= otherTemp.get_temperatureF())
{
return true;
}
else
{
return false;
}}
public boolean islessthan(Temperature otherTemp)
{
if (this.scale == 'C' && this.degree <= otherTemp.get_temperatureC())
{
return true;
}
else if (this.scale == 'F' && this.degree <= otherTemp.get_temperatureF())
{
return true;
}
else
{
return false;
}}
public String toString()
{
return Double.toString(degree) + scale;
}}
---------------------------------------------
package abb1;
class TemperatureDemo {
public static void main(String[] args)
{
Temperature cloudC = new Temperature(0.0, 'C');
Temperature cloudF = new Temperature(32.0, 'F');
Temperature hotC = new Temperature(100.0);
hotC.setScale('C');
Temperature hotF = new Temperature(212.0);
hotF.setScale('F');
Temperature degreeC = new Temperature();
degreeC.setScale(-40.0, 'C');
Temperature degreeF = new Temperature();
degreeF.setScale('F');
degreeF.setdegree( -40.0);
System.out.println("iceC = " + cloudC );
System.out.println("iceF = " + cloudF );
System.out.println("hotC = " + hotC );
System.out.println("hotF = " + hotF );
System.out.println("degreeC = " + degreeC + " , " + degreeC.get_temperatureF() + "F");
System.out.println("degreeF = " + degreeF + " , " + degreeF.get_temperatureC() + "C");
System.out.print("Test isequals");
System.out.println(cloudC + " = " + degreeC + " is " + cloudC.isequals(degreeC));
System.out.println(cloudC + " = " + cloudF + " is " + cloudC.isequals(cloudF));
System.out.println(cloudF + " = " + degreeC + " is " + cloudF.isequals(degreeC));
System.out.println(cloudF + " = " + cloudC + " is " + cloudF.isequals(cloudC));
System.out.print("Test islessthan");
System.out.println(cloudC + " <= " + degreeC + " is " + cloudC.islessthan(degreeC));
System.out.println(cloudC + " <= " + hotF + " is " + cloudC.islessthan(hotF));
System.out.println(cloudF + " <= " + degreeC + " is " + cloudF.islessthan(degreeC));
System.out.println(cloudF + " <= " + hotC + " is " + cloudF.islessthan(hotC));
System.out.print("Test isgreaterthan");
System.out.println(cloudC + " >= " + hotC + " is " +cloudC.isgreaterthan(hotC));
System.out.println(cloudC + " >= " + degreeF + " is " + cloudC.isgreaterthan(degreeF));
System.out.println(cloudF + " >= " + hotF + " is" + cloudF.isgreaterthan(hotF));
System.out.println(cloudF + " >= " + degreeF + " is " + cloudF.isgreaterthan(degreeF));
}}
---------------------------------------------------
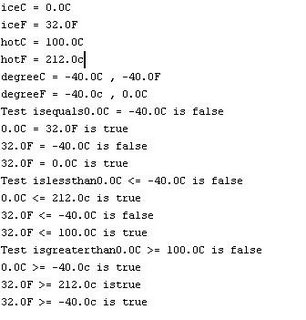
Java Overloading
package sss3;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class Date {
private String month;
private int day;
private int year;
public Date() {
month = "January";
day = 1;
year = 1000;
}
public Date(int monthInt, int day, int year) {
setDate(monthInt, day, year);
}
public Date(String monthString, int day, int year) {
setDate(monthString, day, year);
}
public Date(int year) {
setDate(1, 1, year);
}
public Date(Date aDate) {
if (aDate == null) {
System.out.println("Fatal Error.");
System.exit(0);
}
month = aDate.month;
day = aDate.day;
year = aDate.year;
}
public void setDate(int monthInt, int day, int year) {
if (dateOK(monthInt, day, year)) {
this.month = monthString(monthInt);
this.day = day;
this.year = year;
}
else {
System.out.println("Fatal Error");
System.exit(0);
}
}
public void setDate(String monthString, int day, int year) {
if (dateOK(monthString, day, year)) {
this.month = monthString;
this.day = day;
this.year = year;
}
else {
System.out.println("Fatal Error");
System.exit(0);
}
}
public void setDate(int year) {
setDate(1, 1, year);
}
public void setYear(int year) {
if ( (year < 1000 ) || (year > 9999)) {
System.out.println("Fatal Error");
System.exit(0);
}
else
this.year = year;
}
public void setMonth(int monthNumber) {
if ( (monthNumber <= 0) || (monthNumber > 12)) {
System.out.println("Fatal Error");
System.exit(0);
}
else
month = monthString(monthNumber);
}
public void setDay(int day) {
if ( (day <= 0) || (day > 31)) {
System.out.println("Fatal Error");
System.exit(0);
}
else
this.day = day;
}
public int getMonth() {
if (month.equals("January"))
return 1;
else if (month.equals("February"))
return 2;
else if (month.equalsIgnoreCase("March"))
return 3;
else if (month.equalsIgnoreCase("April"))
return 4;
else if (month.equalsIgnoreCase("May"))
return 5;
else if (month.equals("June"))
return 6;
else if (month.equalsIgnoreCase("July"))
return 7;
else if (month.equalsIgnoreCase("August"))
return 8;
else if (month.equalsIgnoreCase("September"))
return 9;
else if (month.equalsIgnoreCase("October"))
return 10;
else if (month.equals("November"))
return 11;
else if (month.equals("December"))
return 12;
else {
System.out.println("Fatal Error");
System.exit(0);
return 0;
}}
public int getDay() {
return day;
}
public int getYear() {
return year;
}
public String toString() {
return (month + " " + day + ", " + year);
}
public boolean equals(Date otherDate) {
return ( (month.equals(otherDate.month))
&& (day == otherDate.day) && (year == otherDate.year));
}
public boolean precedes(Date otherDate) {
return ( (year < otherDate.year) ||
(year == otherDate.year && getMonth() < otherDate.getMonth()) ||
(year == otherDate.year && month.equals(otherDate.month)
&& day < otherDate.day));
}
public void readInput() throws IOException {
boolean tryAgain = true;
BufferedReader keyboard = new BufferedReader(new InputStreamReader(System.
in));
while (tryAgain) {
System.out.println("Enter month, day, and year.");
System.out.println("Do not use a comma.");
String monthInput = keyboard.readLine();
int dayInput = keyboard.read();
int yearInput = keyboard.read();
if (dateOK(monthInput, dayInput, yearInput)) {
setDate(monthInput, dayInput, yearInput);
tryAgain = false;
}
else
System.out.println("Illegal date. Reenter input.");
}
}
private boolean dateOK(int monthInt, int dayInt, int yearInt) {
return ( (monthInt >= 1) && (monthInt <= 12) &&
(dayInt >= 1) && (dayInt <= 31) &&
(yearInt >= 1000) && (yearInt <= 9999));
}
private boolean dateOK(String monthString, int dayInt, int yearInt) {
return (monthOK(monthString) &&
(dayInt >= 1) && (dayInt <= 31) &&
(yearInt >= 1000) && (yearInt <= 9999));
}
private boolean monthOK(String month) {
return (month.equals("January") || month.equals("February") ||
month.equals("March") || month.equals("April") ||
month.equals("May") || month.equals("June") ||
month.equals("July") || month.equals("August") ||
month.equals("September") || month.equals("October") ||
month.equals("November") || month.equals("December"));
}
private String monthString(int monthNumber) {
switch (monthNumber) {
case 1:
return "January";
case 2:
return "February";
case 3:
return "March";
case 4:
return "April";
case 5:
return "May";
case 6:
return "June";
case 7:
return "July";
case 8:
return "August";
case 9:
return "September";
case 10:
return "October";
case 11:
return "November";
case 12:
return "December";
default:
System.out.println("Fatal Error");
System.exit(0);
return "Error";
}}}
-------------------------------------------------
package sss3;
public class abc {
public static void main(String[] args) {
Date date1 = new Date("December", 16, 1770),
date2 = new Date(1, 27, 1756),
date3 = new Date(1882),
date4 = new Date();
System.out.println("Whose birthday is " + date1 + "?");
System.out.println("Whose birthday is " + date2 + "?");
System.out.println("Whose birthday is " + date3 + "?");
System.out.println("The default date is " + date4 + ".");
} }
------------------------------------------------
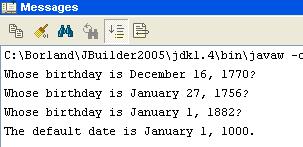
Tuesday, December 05, 2006
Lab Java Constructor
package sss3;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class Date
{
private String month;
private int day;
private int year;
public Date() {
month = "Jan";
day = 1;
year = 1000;
}
public Date(int monthInt, int day, int year) {
setDate(monthInt, day, year);
}
public Date(String monthString, int day, int year) {
setDate(monthString, day, year);
}
public Date(int year) {
setDate(1, 1, year);
}
public Date(Date aDate) {
if (aDate == null) {
System.out.println("Fatal Error.");
System.exit(0);
}
month = aDate.month;
day = aDate.day;
year = aDate.year;
}
public void setDate(int monthInt, int day, int year) {
if (dateOK(monthInt, day, year)) {
this.month = monthString(monthInt);
this.day = day;
this.year = year;
} else {
System.out.println("Fatal Error");
System.exit(0);
}}
public void setDate(String monthString, int day, int year) {
if (dateOK(monthString, day, year)) {
this.month = monthString;
this.day = day;
this.year = year;
} else {
System.out.println("Fatal Error");
System.exit(0);
}}
public void setDate(int year) {
setDate(1, 1, year);
}
public void setYear(int year) {
if ((year < 1000) || (year > 9999)) {
System.out.println("Fatal Error");
System.exit(0);
} else {
this.year = year;
}}
public void setMonth(int monthNumber) {
if ((monthNumber <= 0) || (monthNumber > 12)) {
System.out.println("Fatal Error");
System.exit(0);
} else {
month = monthString(monthNumber);
}}
public void setDay(int day) {
if ((day <= 0) || (day > 31)) {
System.out.println("Fatal Error");
System.exit(0);
} else {
this.day = day;
}}
public int getMonth() {
if (month.equals("Jan")) {return 1;
} else if (month.equals("Feb")) {return 2;
} else if (month.equals("Mar")) {return 3;
} else if (month.equals("Apr")) {return 4;
} else if (month.equals("May")) {return 5;
} else if (month.equals("Jun")) {return 6;
} else if (month.equals("Jul")) {return 7;
} else if (month.equals("Aug")) {return 8;
} else if (month.equals("Sep")) {return 9;
} else if (month.equals("Oct")) {return 10;
} else if (month.equals("Nov")) {return 11;
} else if (month.equals("Dec")) {return 12;
} else {
System.out.println("Fatal Error");
System.exit(0);
return 0; //Needed to keep the compiler happy
}}
public int getDay() {
return day;
}
public int getYear() {
return year;
}
public String toString() {
return (month + " " + day + ", " + year);
}
public boolean equals(Date otherDate) {
return ((month.equals(otherDate.month))
&& (day == otherDate.day) && (year == otherDate.year));
}
public boolean precedes(Date otherDate) {
return ((year < otherDate.year) ||
(year == otherDate.year && getMonth() < otherDate.getMonth()) ||
(year == otherDate.year && month.equals(otherDate.month)
&& day < otherDate.day));
}
public void readInput() throws IOException {
boolean tryAgain = true;
BufferedReader keyboard = new BufferedReader(
new InputStreamReader(System.in));
while (tryAgain) {
System.out.println(
"Enter month, day, and year on three lines.");
System.out.println(
"Enter month, day, and year as three integers.");
int monthInput = Integer.parseInt(keyboard.readLine());
int dayInput = Integer.parseInt(keyboard.readLine());
int yearInput = Integer.parseInt(keyboard.readLine());
if (dateOK(monthInput, dayInput, yearInput)) {
setDate(monthInput, dayInput, yearInput);
tryAgain = false;
} else {
System.out.println("Illegal date. Reenter input.");
}}}
private boolean dateOK(int monthInt, int dayInt, int yearInt) {
return ((monthInt >= 1) && (monthInt <= 12) &&
(dayInt >= 1) && (dayInt <= 31) &&
(yearInt >= 1000) && (yearInt <= 9999));
}
private boolean dateOK(String monthString, int dayInt, int yearInt) {
return (monthOK(monthString) &&
(dayInt >= 1) && (dayInt <= 31) &&
(yearInt >= 1000) && (yearInt <= 9999));
}
private boolean monthOK(String month) {
return (month.equals("Jan") || month.equals("Feb") ||
month.equals("Mar") || month.equals("Apr") ||
month.equals("May") || month.equals("Jun") ||
month.equals("Jul") || month.equals("Aug") ||
month.equals("Sep") || month.equals("Oct") ||
month.equals("Nov") || month.equals("Dec"));
}
private String monthString(int monthNumber) {
switch (monthNumber) {
case 1:return "Jan";
case 2:return "Feb";
case 3:return "Mar";
case 4:return "Apr";
case 5:return "May";
case 6:return "Jun";
case 7:return "Jul";
case 8:return "Aug";
case 9:return "Sep";
case 10:return "Oct";
case 11:return "Nov";
case 12:return "Dec";
default:
System.out.println("Fatal Error");
System.exit(0);
return "Error";
}}}
-----------
package sss3;
class abc {
public static void main(String[] args) {
Date date1 = new Date("Jan", 1, 2000), date2 = new Date("Feb", 1, 2000),
date3 = new Date("Feb" , 1 , 2000), date4 = new Date("Mar" , 1 , 2000);
System.out.println( date1 );
System.out.println( date2 );
System.out.println( date3);
System.out.println( date4);
}}
----------------
Jan 1, 2000
Feb 1, 2000
Feb 1, 2000
Mar 1, 2000
Tuesday, November 28, 2006
Lab Class Definitions III
package untitled2;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class DateFifthTry
{
private String month;
private int day;
private int year;
public String toString() {
return (month + " " + day + ", " + year);
}
public void writeOutput() {
System.out.println(month + " " + day + ", " + year);
}
public boolean equals(DateFifthTry otherDate) {
return (month.equals(otherDate.month) && day == (otherDate.day) &&
year == (otherDate.year));
}
public boolean precedes(DateFifthTry otherDate) {
return (year < otherDate.year) ||
(year == otherDate.year && getMonth() < otherDate.getMonth()) ||
(year == otherDate.year && month.equals(otherDate.month)&& day < otherDate.day);
}
public int getMonth() {
if (month.equals("Jan")) {
return 1;} else if (month.equals("Feb")) {
return 2;} else if (month.equals("Mar")) {
return 3;} else if (month.equals("Apr")) {
return 4;} else if (month.equals("May")) {
return 5;} else if (month.equals("Jun")) {
return 6;} else if (month.equals("Jul")) {
return 7;} else if (month.equals("Aug")) {
return 8;} else if (month.equals("Sep")) {
return 9;} else if (month.equals("Oct")) {
return 10;} else if (month.equals("Nov")) {
return 11;} else if (month.equals("Dec")) {
return 12;} else {System.out.println("Fateal Error");
System.exit(0);
return 0;
}}
public String monthString(int monthNmmber) {
switch (monthNmmber) {
case 1:return "Jan";
case 2:return "Feb";
case 3:return "Mar";
case 4:return "Apr";
case 5:return "May";
case 6:return "Jun";
case 7:return "Jul";
case 8:return "Aug";
case 9:return "Sep";
case 10:return "Oct";
case 11:return "Nov";
case 12:return "Dec";
default:
System.out.println("Fateal Error");
System.exit(0);
return "Error";
}}
public void setDate(int newMonth, int newDay, int newYear) {
month = monthString(newMonth);
day = newDay;
year = newYear;
}}
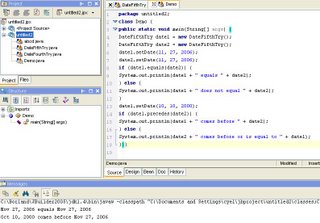